Project Overview
The Python Flask REST API project hosted at GitHub Repository is a foundational REST API developed using Flask, designed for handling and serving JSON data over HTTP requests. This project showcases essential REST API functionalities, enabling users to perform CRUD operations for managing a sample dataset. It’s an ideal starting point for those learning API development or building lightweight, scalable backend services.
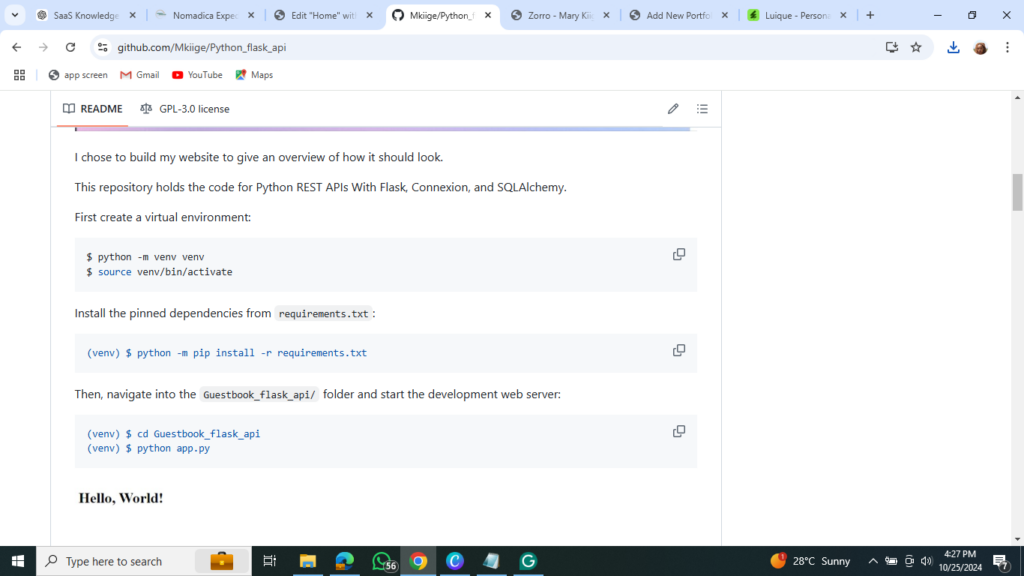
Project Goals
- Implement a Basic REST API: Create an easy-to-understand API structure using Flask that covers CRUD operations and follows RESTful design principles.
- Handle JSON Data Effectively: Enable data serialization and deserialization in JSON format, making it easy to integrate with frontend applications.
- Ensure Clean Code Structure and Modular Design: Organize the codebase with modular components, making it maintainable and scalable.
- Provide Secure and Error-Handled Endpoints: Include basic authentication and error handling to ensure safe and reliable API responses.
Features and Functionalities
- CRUD Operations: Supports Create, Read, Update, and Delete actions on a sample dataset, demonstrating core REST API functionality.
- JSON Data Serialization: Handles JSON request and response data, allowing seamless data integration with other applications.
- Error Handling: Provides consistent error responses to enhance user experience and facilitate debugging.
- Basic Authentication: Includes basic user authentication to restrict access to certain endpoints, showcasing initial security practices.
- Modular Structure: Organized code structure with separate modules for routes, models, and configurations, making the API scalable.
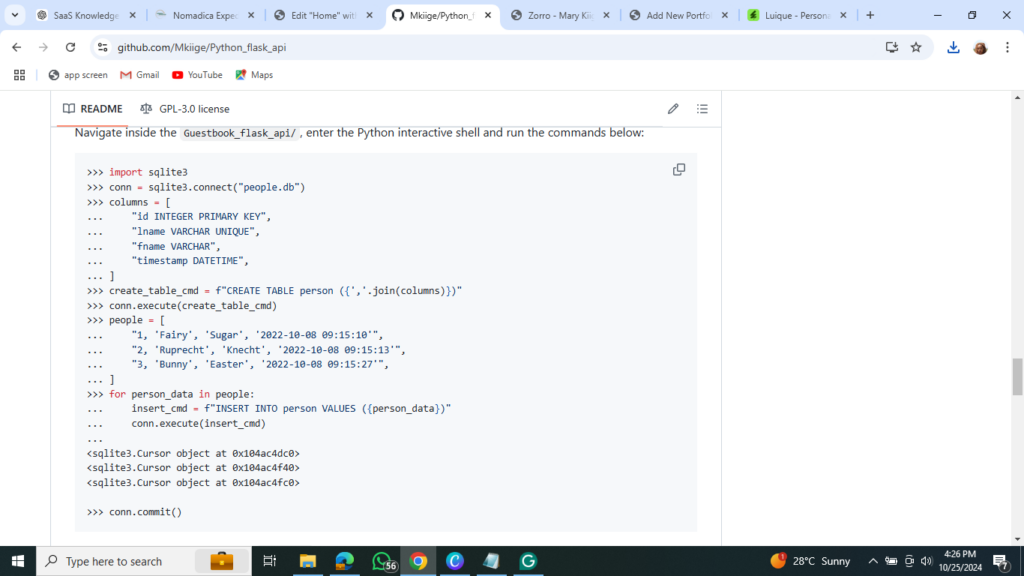
Technical Stack
- Framework: Flask for lightweight, easy-to-deploy API structure.
- Database: SQLite for quick data storage and retrieval, suitable for prototyping and development.
- Authentication: Basic authentication for securing endpoints.
- Testing: Unit tests for endpoint validation, ensuring each route performs as expected.
Project Phases
- API Planning and Setup: Define the dataset and create a plan for the CRUD operations, setting up Flask and configuring the environment.
- Data Modeling and CRUD Route Implementation: Develop models using SQLAlchemy (if implemented) and configure CRUD routes for data interaction.
- Authentication and Error Handling: Add basic authentication for restricted routes and implement error handlers for consistent response formatting.
- Testing and Documentation: Write unit tests to verify endpoint functionality and document the API for easy integration.
- Optimization and Deployment: Optimize response times and deploy on a platform such as Heroku for accessible demonstration.
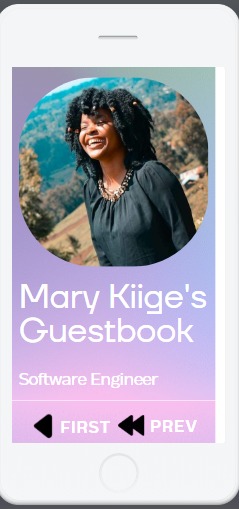
Challenges and Solutions
- Challenge: Simplifying API for Beginners Without Compromising Quality.
- Solution: Used Flask’s minimalistic approach to demonstrate core concepts without overwhelming complexity, focusing on essential API patterns.
- Challenge: Providing a Structured and Scalable Codebase.
- Solution: Organized the code using blueprints and modular structure, laying a foundation that can be expanded for larger projects.
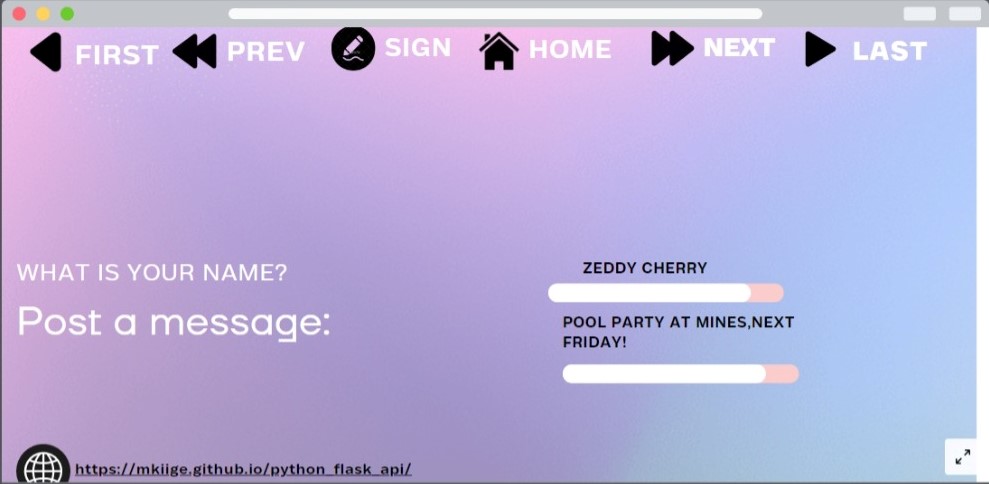
Outcome
- Educational Value: This project serves as a hands-on learning experience for new developers, showcasing the essentials of REST API development with Flask.
- Extensibility: The structured codebase allows for easy expansion, whether adding new features or integrating with other services.
- Foundation for Further Development: Offers a base that can be enhanced with additional features like JWT authentication, advanced error handling, or integration with databases like PostgreSQL.
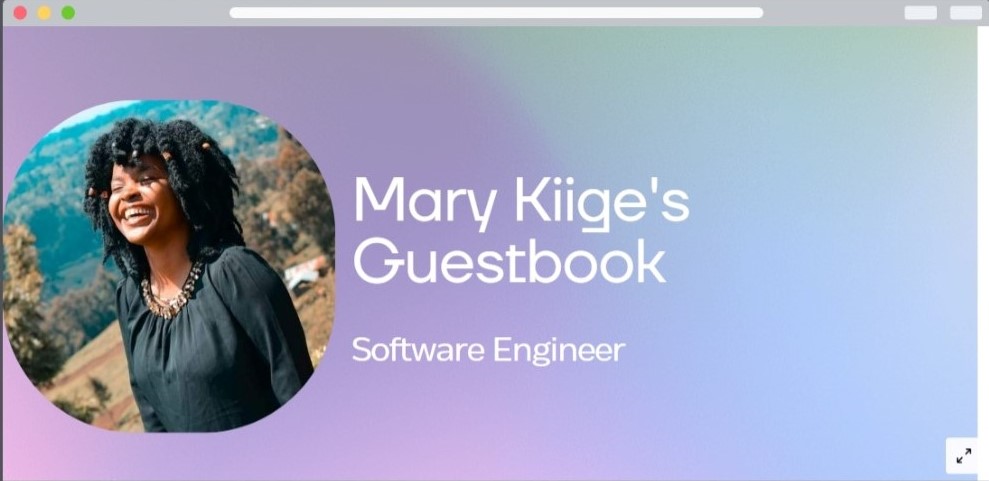